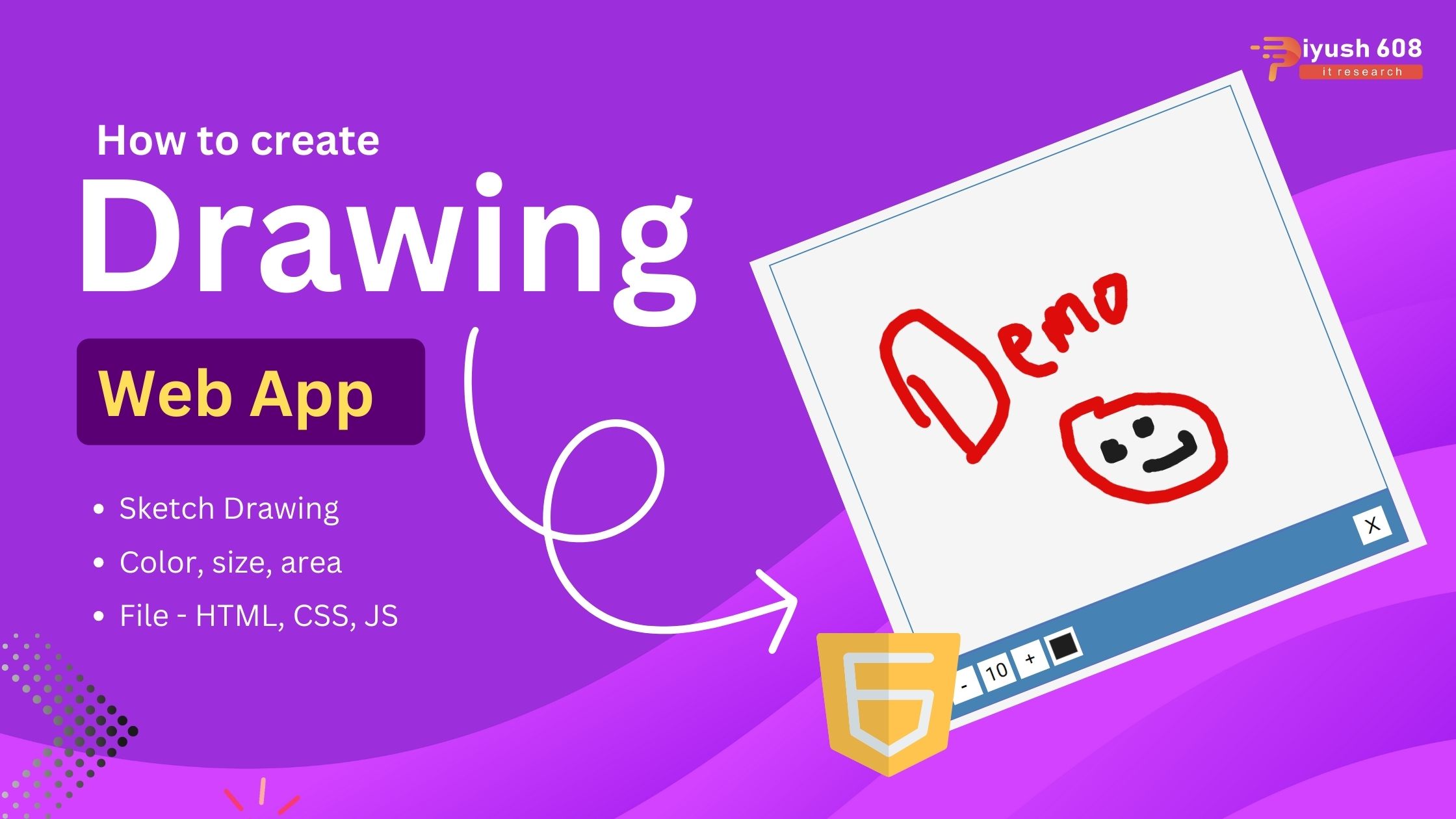
Creating a drawing app using HTML, CSS, and JavaScript involves using the HTML5 <canvas>
element for drawing and JavaScript to handle the drawing logic. Below is a simple implementation:
HTML (index.html)
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <link rel="stylesheet" href="style.css" /> <title>Drawing App</title> </head> <body> <canvas id="canvas" width="800" height="700"></canvas> <div class="toolbox"> <button id="decrease">-</button> <span id="size">10</span> <button id="increase">+</button> <input type="color" id="color"> <button id="clear">X</button> </div> <script src="script.js"></script> </body> </html>
CSS (styles.css)
@import url('https://fonts.googleapis.com/css2?family=Roboto:wght@400;700&display=swap'); * { box-sizing: border-box; } body { background-color: #f5f5f5; font-family: 'Roboto', sans-serif; display: flex; flex-direction: column; align-items: center; justify-content: center; height: 100vh; margin: 0; } canvas { border: 2px solid steelblue; } .toolbox { background-color: steelblue; border: 1px solid slateblue; display: flex; width: 804px; padding: 1rem; } .toolbox > * { background-color: #fff; border: none; display: inline-flex; align-items: center; justify-content: center; font-size: 2rem; height: 50px; width: 50px; margin: 0.25rem; padding: 0.25rem; cursor: pointer; } .toolbox > *:last-child { margin-left: auto; }
JavaScript (scripts.js)
const canvas = document.getElementById('canvas'); const increaseBtn = document.getElementById('increase'); const decreaseBtn = document.getElementById('decrease'); const sizeEL = document.getElementById('size'); const colorEl = document.getElementById('color'); const clearEl = document.getElementById('clear'); const ctx = canvas.getContext('2d'); let size = 10 let isPressed = false colorEl.value = 'black' let color = colorEl.value let x let y canvas.addEventListener('mousedown', (e) => { isPressed = true x = e.offsetX y = e.offsetY }) document.addEventListener('mouseup', (e) => { isPressed = false x = undefined y = undefined }) canvas.addEventListener('mousemove', (e) => { if(isPressed) { const x2 = e.offsetX const y2 = e.offsetY drawCircle(x2, y2) drawLine(x, y, x2, y2) x = x2 y = y2 } }) function drawCircle(x, y) { ctx.beginPath(); ctx.arc(x, y, size, 0, Math.PI * 2) ctx.fillStyle = color ctx.fill() } function drawLine(x1, y1, x2, y2) { ctx.beginPath() ctx.moveTo(x1, y1) ctx.lineTo(x2, y2) ctx.strokeStyle = color ctx.lineWidth = size * 2 ctx.stroke() } function updateSizeOnScreen() { sizeEL.innerText = size } increaseBtn.addEventListener('click', () => { size += 5 if(size > 50) { size = 50 } updateSizeOnScreen() }) decreaseBtn.addEventListener('click', () => { size -= 5 if(size < 5) { size = 5 } updateSizeOnScreen() }) colorEl.addEventListener('change', (e) => color = e.target.value) clearEl.addEventListener('click', () => ctx.clearRect(0,0, canvas.width, canvas.height))
Reviews
There are no reviews yet. Be the first one to write one.